10 Common Mistakes to Avoid When Developing Apps on React
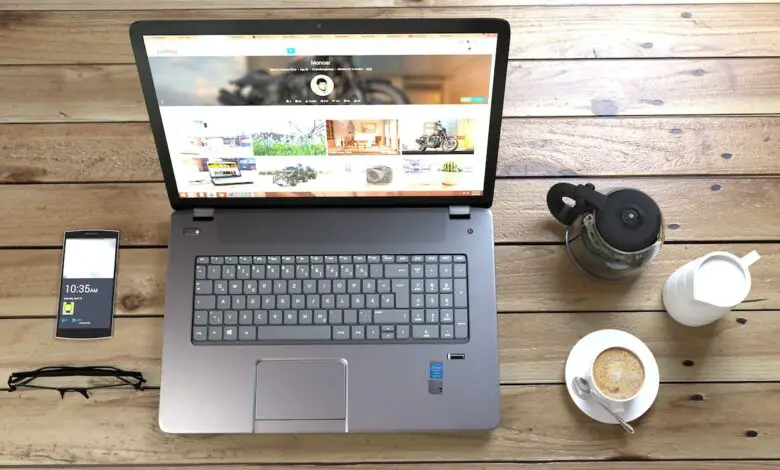
One of the most widely used JavaScript libraries for UI development is React. Its component-based architecture and efficient rendering make it a developer favorite. However, like any technology, React has its pitfalls. In this article, we’ll look at common mistakes that developers make when working with React, and give tips on how to avoid them.
Also, the best way to avoid any mistakes is to turn to professionals from Keenethics web development company and trust your resources to them.
Mistake 1: Not Understanding the Lifecycle of a Component
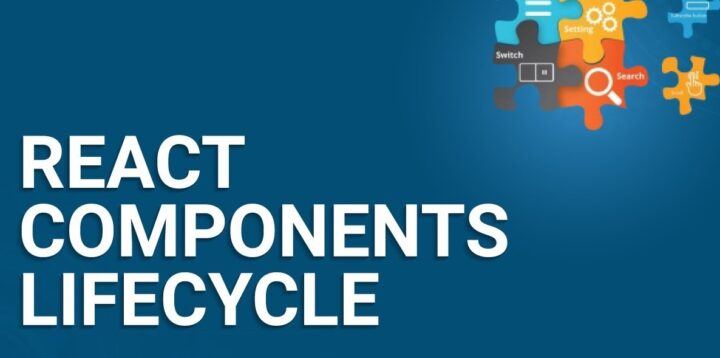
React components have a lifecycle that includes various stages such as mounting, updating, and unmounting. Each stage provides lifecycle methods that allow developers to perform specific actions at specific stages. The first of the common mistakes is the lack of understanding of this life cycle and the incorrect use of these methods.
For instance, a developer can inadvertently utilize the componentDidMount method rather than the componentDidUpdate method to asynchronously obtain data. Performance problems and pointless API requests may result from this.
To avoid this mistake, take the time to learn and understand the lifecycle of a React component. Read the official documentation and view tutorials that cover this topic in detail. Learn about each lifecycle method and its correct use cases. You can create React components that are more effective and error-free by understanding the lifecycle.
Mistake 2: Immediate Change of State
State in React is a key concept that allows components to manage and update their data. However, changing state directly is a common mistake that can lead to unpredictable behavior and difficult debugging problems.
For example, if a developer directly modifies a state object using mutation methods such as push or splice, React will not detect the change. This can cause the component’s rendering to not update after a state change, resulting in an inconsistent UI.
The state is a critical aspect of React, and it’s very important to use it correctly. Be sure to avoid changing the state directly and use setState instead. Using a large state object can lead to performance issues in your React application. Be sure to break the state into smaller, more manageable chunks.
Also, remember that React state must be immutable. Instead of changing the state directly, create new objects or arrays based on the existing state and update them accordingly.
Mistake 3: Improper Use of Conditional Rendering
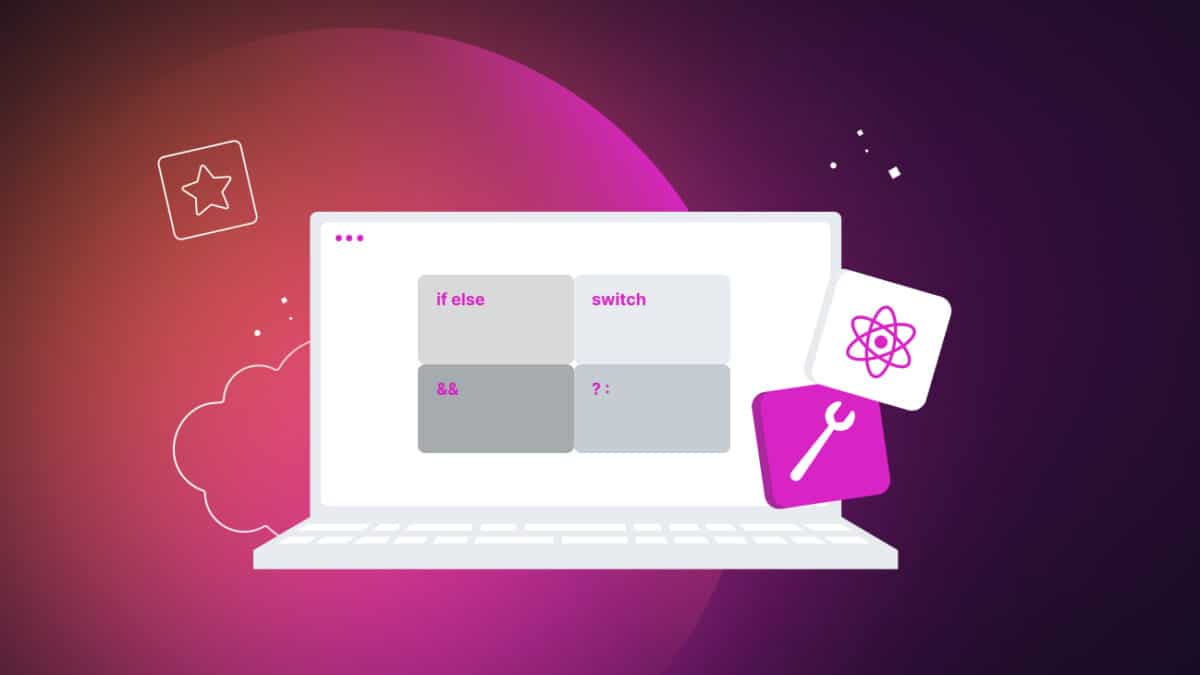
React’s strong conditional rendering functionality enables components to show various information depending on specific circumstances. However, using conditional rendering incorrectly can lead to code that is difficult to understand and maintain.
One of the common mistakes is the excessive nesting of ternary operators or the use of complex logical expressions in JSX. This can make the code difficult to read and debug, especially when the number of conditions increases.
To avoid this error, consider other approaches, such as component composition or offloading conditional rendering logic to individual functions. This will help keep your JSX clean and readable. Also, consider using helper functions or libraries, such as class names or classes, to manage classes and styles in a more declarative and maintainable way.
Mistake 4: Inefficient Rendering
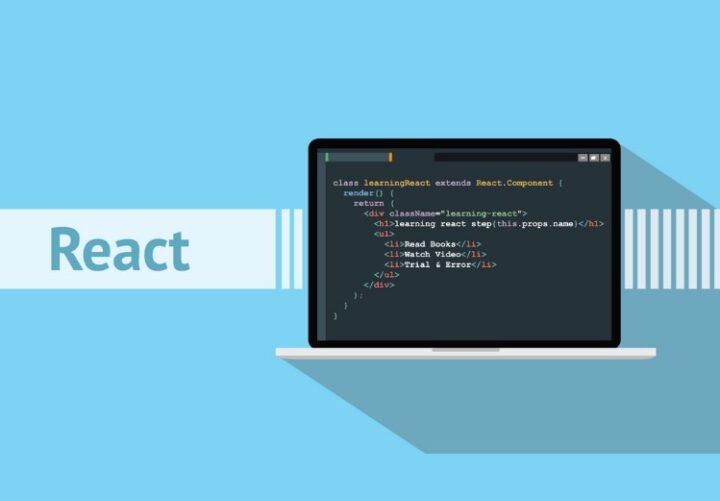
Efficient rendering is one of React’s main strengths, but it can be undermined by bad programming practices. Rendering redundant components or calling unnecessary re-renders can negatively affect the performance of your React application.
One of the common causes of inefficient rendering is binding event handlers directly in the render method. This causes a new instance of the function to be created each time the component is rendered, causing unnecessary re-renders of child components.
To optimize rendering performance, bind event handlers in the constructor or use arrow functions because they are lexically scoped and do not create new instances on each render. Also, consider using a higher-level React.memo component or the React.PureComponent class to avoid unnecessary re-rendering of components that haven’t changed.
Mistake 5: Incorrect Use of Keys
In React, keys are used so that React itself can identify which items in a list have been changed, added, or removed. However, using them incorrectly can lead to strange errors and unpredictable behavior.
A common mistake is to use array indices as keys when rendering dynamic lists. This can lead to problems when the order of elements changes, leading to incorrect rendering and possible data loss.
To use keys efficiently, make sure that each key is unique among its neighbors. If possible, use persistent identifiers, such as unique identifiers for keys. Avoid using index numbers as keys, especially if the list is dynamically sorted or filtered. By providing persistent and unique keys, you allow React to efficiently update components and maintain the correct state of components.
Mistake 6: Not Using PureComponent or shouldComponentUpdate, React.memo
Using PureComponent or shouldComponentUpdate, React.memo can greatly improve the performance of your React application by preventing unnecessary re-rendering. Be sure to use them when appropriate.
Mistake 7: Not Using CSS Modules or Styled-components
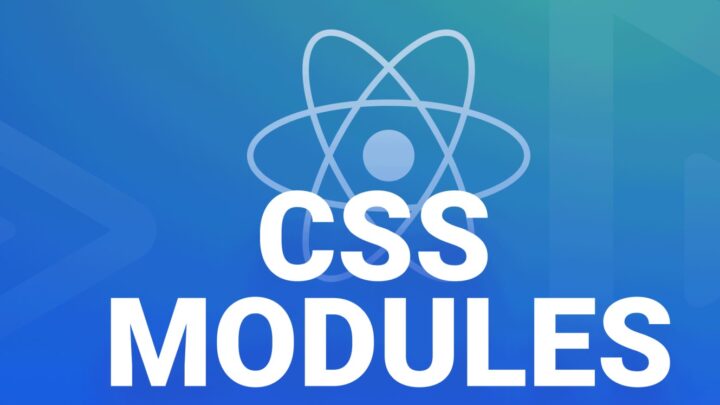
CSS modules and styled-components can help you prevent CSS conflicts and make your code easier to manage. Be sure to use them when appropriate.
Mistake 8: Incorrect Use of Event Handlers
Event handlers are used in React to handle user interaction, and it is very important to use them correctly. Make sure you bind event handlers correctly and avoid arrow functions in render methods.
Mistake 9: Not Using React DevTools
React DevTools can help you debug and optimize your React application, and it’s important to use them. Be sure to install and use the React DevTools extension.
Mistake 10: Not Using the Correct Version of React
Using an outdated version of React can lead to unexpected behavior and security breaches. Be sure to use the latest stable version of React.
In this article, we’ve looked at the most common mistakes developers make when working with React and provided tips on how to avoid them. Understanding the lifecycle of components, avoiding direct state changes, using conditional rendering correctly, optimizing rendering performance, and using list keys are important aspects of React development.